Getting Started
Quick Start
npx create-razzle-app@three my-appcd my-appnpm start
Then open http://localhost:3000/ to see your app. Your console should look like this:

That's it. You don't need to worry about setting up multiple webpack configs or other build tools. Just start editing src/App.js
and go!
Commands
Below is a list of commands you will probably find useful.
npm start
Runs the project in development mode.
You can view your application at http://localhost:3000
The page will reload if you make edits.
npm run build
Builds the app for production to the build folder.
The build is minified and the filenames include the hashes. Your app is ready to be deployed!
npm run start:prod
Runs the compiled app in production.
You can again view your application at http://localhost:3000
npm test
Runs the test watcher (Jest) in an interactive mode. By default, runs tests related to files changed since the last commit.
npm export
Exports a static version of the application in production mode. Must add the export
command to package.json
's scripts along with a static_export.js
file in the src
directory. See Static Site Generation for more details.
rs
If your application is running, and you need to manually restart your server, you do not need to completely kill and rebundle your application. Instead you can just type rs
and press enter in terminal.
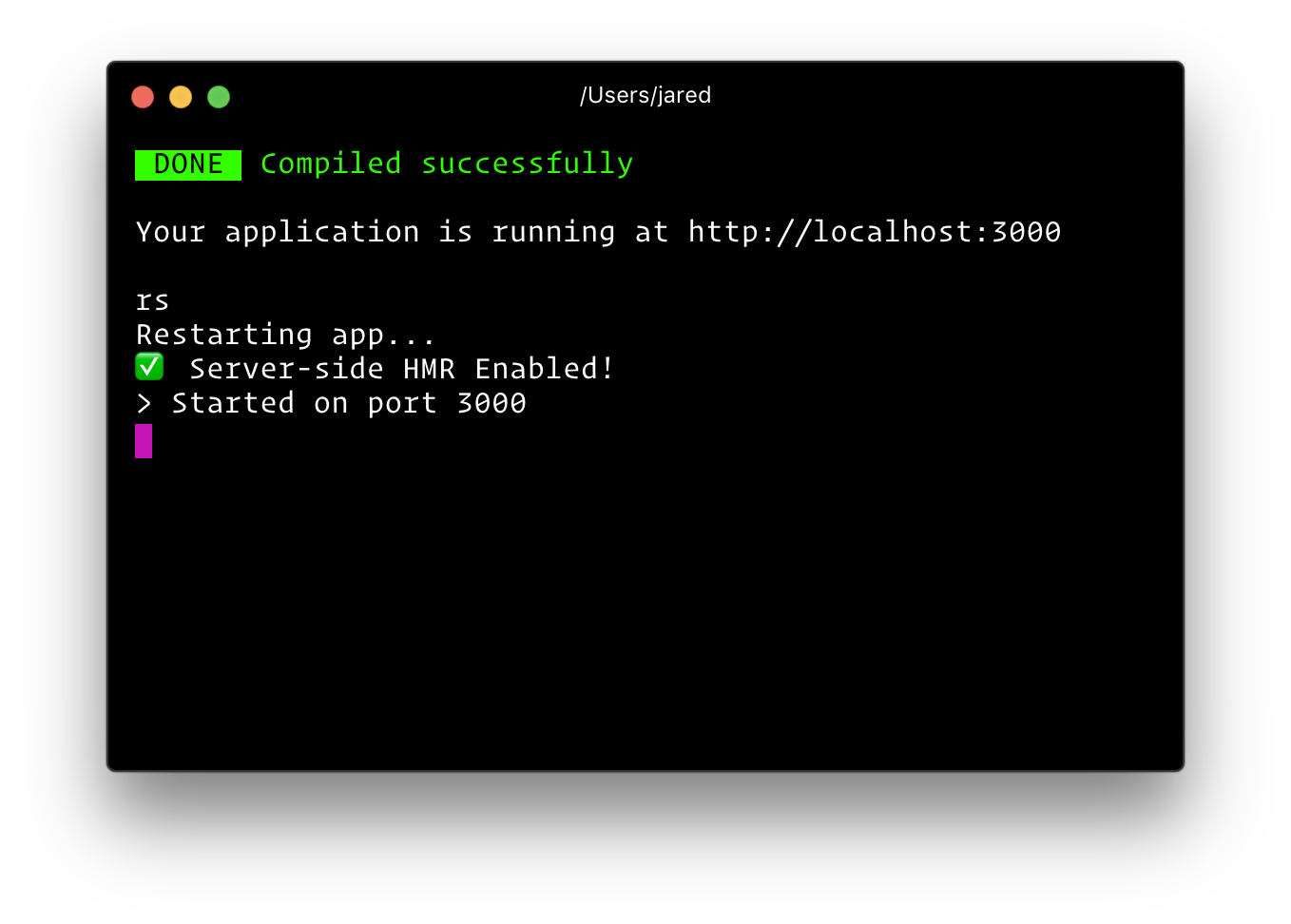
Common issues
If you get a error like this:
node_modules/react-images-upload/index.css:1
.fileUploader {
^
SyntaxError: Unexpected token '.'
at wrapSafe (internal/modules/cjs/loader.js:1072:16)
It means node tries to use index.css
as a node module.
To fix this make sure the module with the css is not externalized.
// razzle.config.js'use strict';module.exports = { modifyWebpackOptions({ options: { webpackOptions, // the modified options that was used to configure webpack/ webpack loaders and plugins } }) { webpackOptions.notNodeExternalPatterns = [/react-images-upload/]; // If you use experimental.newExternals webpackOptions.notNodeExternalResMatch = (request, context) => { return /react-images-upload/.test(request) }; return webpackOptions; },};
Debugging with Inspector
npm start -- --inspect=[host:port]
This will start the node server and enable the inspector agent. The=[host:port]
is optional and defaults to=127.0.0.1:9229
. For more information, see this.npm start -- --inspect-brk=[host:port]
This is the same as--inspect
, but will also break before user code starts. (to give a debugger time to attach before early code runs) For more information, see this.